This blog post will show you how to use the Azure Identity Client library in VB.Net and C# to use a Managed Identity to access a secret in KeyVault. This is assuming that you already have a keyVault secret and the user has the proper access policy to read a keyvault secret. This post will not show you how to do those tasks, only how to implement the Azure Identity client library in a vb.net application and access a secret as well as generating an access token. I have based this blog post on the following documents:
You can find the VB.Net sample in my GitHub here. Also, there is a C# version of the sample in my Github here.
Before we start, lets set up a resource, a system assigned identity for that resource, and then a user managed identity that has access to the resource. The resource that I will set up is simply a VM in Azure ( so I can run visual studio there and have my code work ). The key to this is the code must run in the resource you have configured in order to be able to use the managed identity. You cannot run a desktop app from your local machine and have it use a managed identity in an Azure VM, for example. So, I have setup a VM in Azure with a pretty basic plan ( 8 gb memory ) so that I can install the community edition of Visual Studio and compile and run my code.
The next step is to create the system assigned managed identity. This is pretty straight forward, after creating the VM, go to the “Identity” blade on the VM and the under System assigned, just click the status to “On” and the object ID will be listed for that identity.
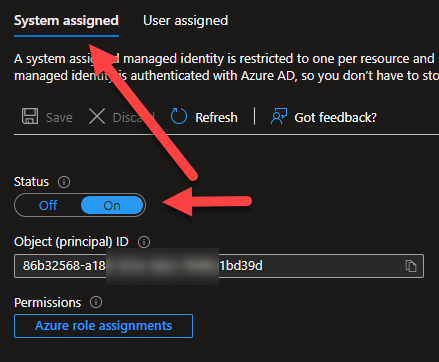
We will be coming back here later to assign our User assigned identity to this resource ( the VM ). To create a user assigned credential, you will need to go to the Managed Identities blade in the portal. Click on the “+ Create” button.
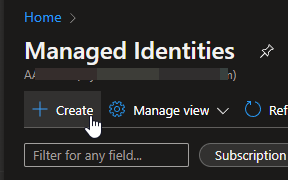
You will need to select the subscription to use, the Resource group, region and name ( the name is going to be the name for your Managed Identity ).
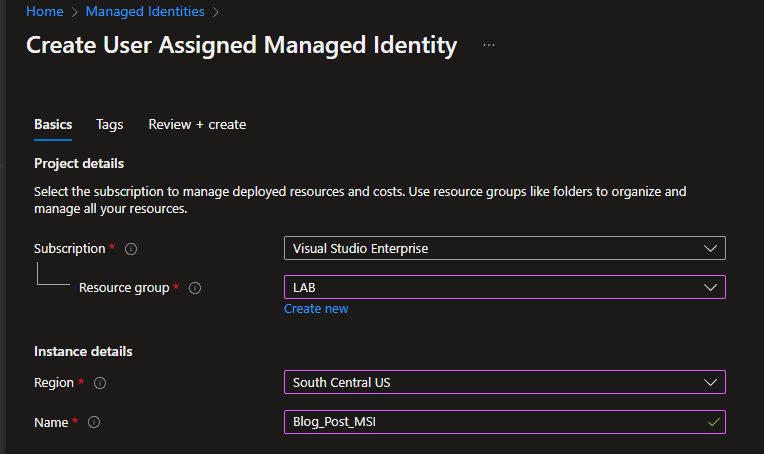
You can then continue to add tags or simply press the Review + Create button. You will need to consent but then the MSI will be created. Once created, click the “Go to resource” button and you will be able to get the Client ID on that blade, which will be needed for the User Assigned Managed Id in code:
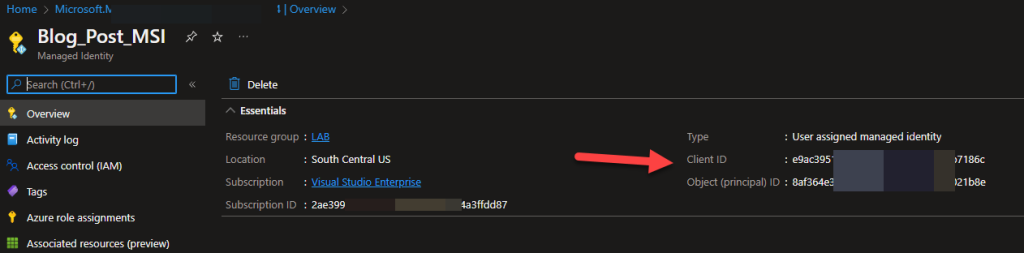
We are not quite done with the User assigned id because you still need to assign it to both the VM ( or where the code is going to run from ) and also create an access policy in KeyVault for both of our MSI’s. To assign the User assigned MSI, go to the VM, click on the User assigned tab, and then add the MSI here.
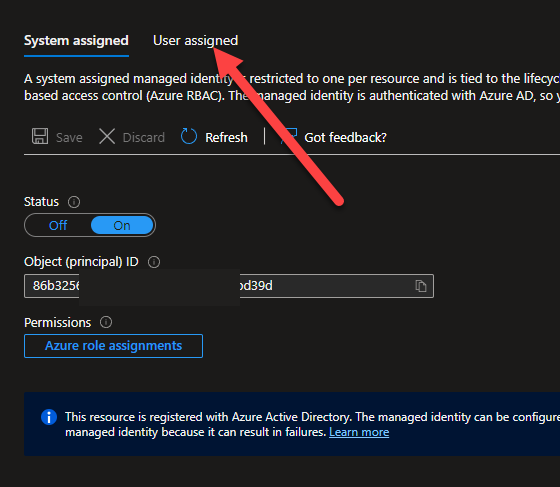
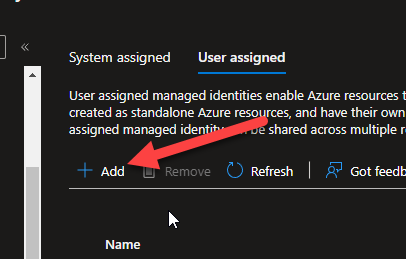
You will be able to find the User assigned managed identity, then select it, click add and it will be added.
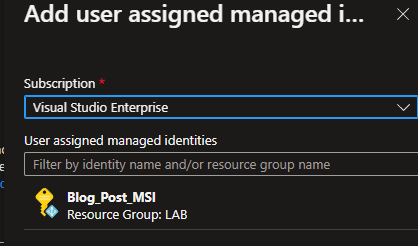
Now, both of our managed identities ( the system assigned and the user assigned ) need to have an access policy in KeyVault created. So, lets go to the KeyVault resource in Azure ( assuming you already have one created and a secret added )..Click the KeyVault, then go to Access policies:
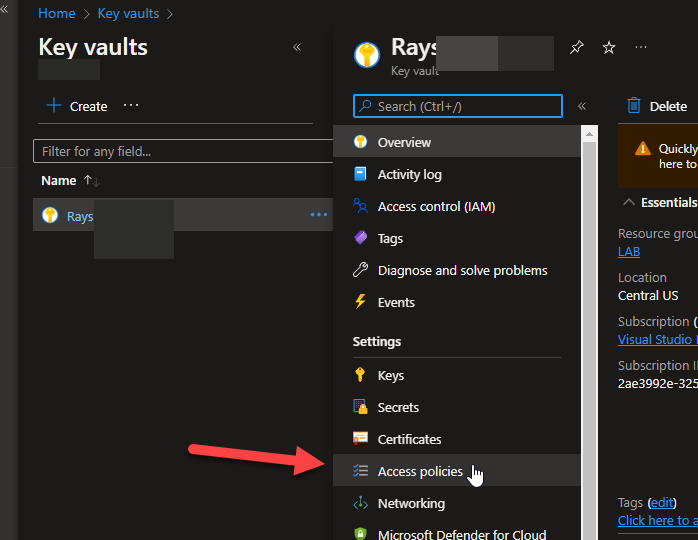
Then, you will need to click the “+ Add Access Policy” to create a new policy for our MSI’s. We need the Get for Key and Secret permissions for this blog:
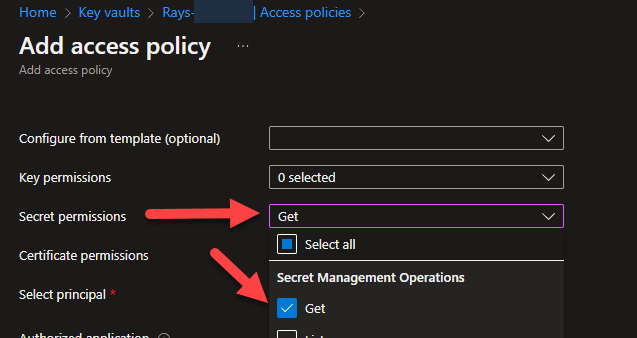
Next, we need to click on the “Select Principal” to add our MSI’s ( you can search by name ) — you can only add one at a time. You should see each MSI listed in the Access Policies blade now. If not, then it hasn’t been added and won’t have access to the keyvault:
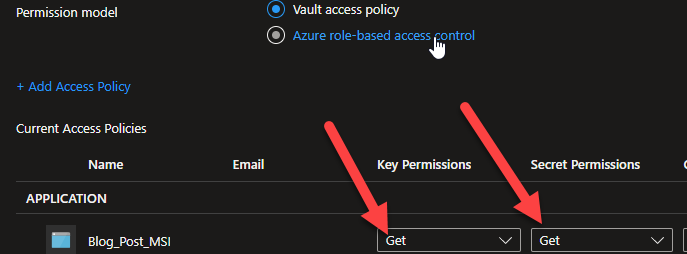
Now for the code: to start, you will need to install the following nuget libraries along with the dependencies that are automatically installed when you choose the packages:
- Azure.Identity
- Azure.Security.KeyVault.Secrets
You will then need the following Imports at the top of your code file:
- Imports Azure.Core
- Imports Azure.Identity
- Imports Azure.Security.KeyVault.Secrets
The key to using the Managed Identities ( system assigned or user assigned ) is setting a ManagedIdentityCredential. For a system assigned one, you just need to set a credential variable to a New ManagedIdentityCredential. For a user assigned credential, you will need to set the credential variable to a New ManagedIdentityCredential( id ) with the id = to the client id for the user assigned credential.
Sub Get_Secret_With_UserAssigned_MSI()
Console.WriteLine($"{vbCrLf}Getting secret with user assigned msi:")
Dim credential As New ManagedIdentityCredential(userAssignedClientId)
Dim client As SecretClient = New SecretClient(keyVaultUri, credential)
Dim secret As KeyVaultSecret = client.GetSecret(keyVaultSecretName).Value
Console.WriteLine($"KeyVault Secret = {secret.Value}{vbCrLf}")
End Sub
Sub Get_Secret_With_SystemAssigned_MSI()
Console.WriteLine($"{vbCrLf}Getting secret with system assigned msi:")
Dim credential As New ManagedIdentityCredential()
Dim client As SecretClient = New SecretClient(keyVaultUri, credential)
Dim secret As KeyVaultSecret = client.GetSecret(keyVaultSecretName).Value
Console.WriteLine($"KeyVault Secret = {secret.Value}{vbCrLf}")
End Sub
The equivalent in C#:
static void Get_Secret_With_UserAssigned_MSI()
{
Console.WriteLine($"\nGetting secret with user assigned msi:");
ManagedIdentityCredential credential = new ManagedIdentityCredential(userAssignedClientId);
SecretClient client = new SecretClient(keyVaultUri, credential);
KeyVaultSecret secret = client.GetSecret(keyVaultSecretName).Value;
Console.WriteLine($"KeyVault Secret = {secret.Value}\n");
}
static void Get_Secret_With_SystemAssigned_MSI()
{
Console.WriteLine($"\nGetting secret with system assigned msi:");
ManagedIdentityCredential credentail = new ManagedIdentityCredential();
SecretClient client = new SecretClient(keyVaultUri, credentail);
KeyVaultSecret secret = client.GetSecret(keyVaultSecretName).Value;
Console.WriteLine($"KeyVault secret = {secret.Value}\n");
}
Download both samples from my GitHub ( linked at the beginning of this blog ) and plug in your MSI information and give it a try after configuring the resource, the keyvault and the MSI’s! In both samples, you will need to change the values for the variables userAssignedClientId, keyVaultSecretName and keyVaultUri to match your environment.
Sample Output:
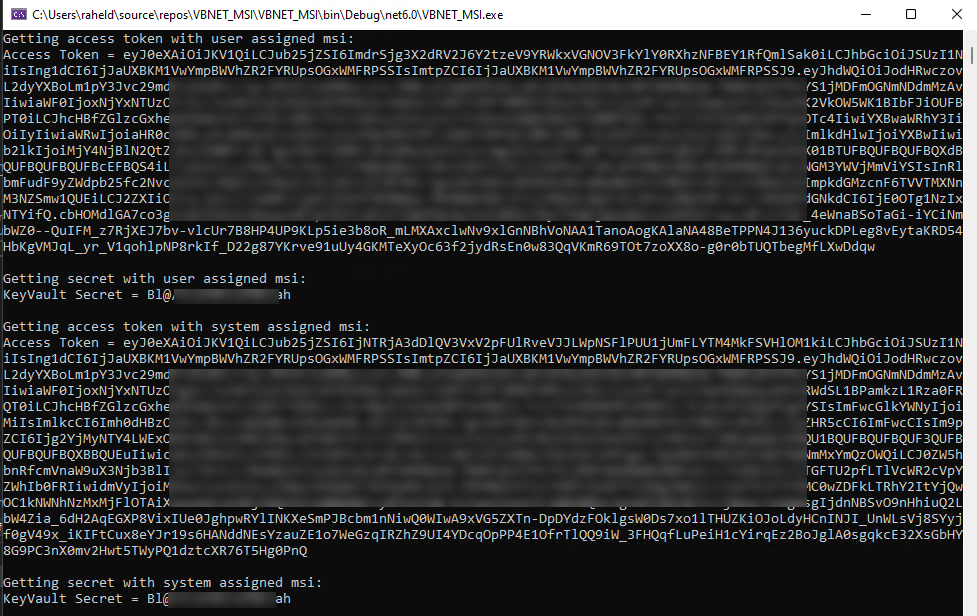
For more reading, see this document about the Managed Identities — there is also a good video on that page.