In this blog, we will use Azure SDK for .NET to perform the following tasks:
- Create an Azure Active Directory (Azure AD) user, query for the created user, and delete the user.
- Create an Azure AD group, query for the created group, and delete the group.
- Add a user to the group’s members.
- Create an Role-based Access Control (RBAC) Role Assignment: we will assign the group created above ‘Storage Blob Data Reader’ role to an Azure Storage Blob container.
Note: RBAC Role Assignment is performed via Azure Resource Manager (ARM) REST endpoint.
As of version 1.33.0, Azure Management Libraries for .NET uses ADAL.NET to authenticate to Azure AD using Client Credentials Grant Flow via the legacy V1 endpoint. It also uses Azure Active Directory Graph (https://graph.windows.net) to query Azure AD Directory Objects.
Note: In general we encourage customers to use the feature-rich MSAL.NET library to authenticate to Azure AD instead of ADAL.NET library. Furthermore using Microsoft Graph REST endpoint (https://graph.microsoft.com) is recommended over the legacy Azure AD Graph (https://graph.windows.net) REST endpoint for managing Azure AD Directory objects.
Prerequisites:
- Create an Application Registration in Azure AD. Configure Azure Active Directory Graph Application permission: Directory.ReadWrite.All, and grant Admin Consent to the configured permissions. This permission is needed to create user and group objects. You also need to create a client secret from the “Certificates & secrets” blade.
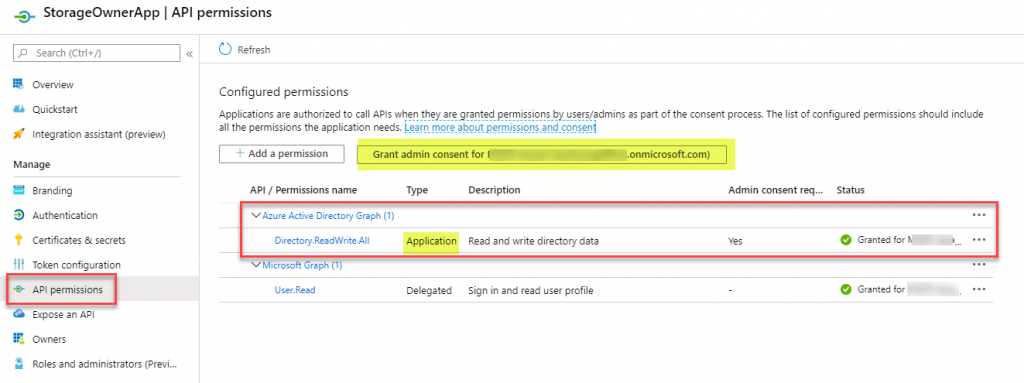
- Add this Application (really Service Principal) to Azure AD’s ‘User Administrator‘ role. This role is essential for deleting the user and group object since the Application Permission Directory.ReadWrite.All does not include user and group deletion per documentation. From the Azure portal, select ‘Azure Active Directory’ -> Roles and Administrators -> User Administrator -> Assignments -> Add Assignment -> add your application to this role.
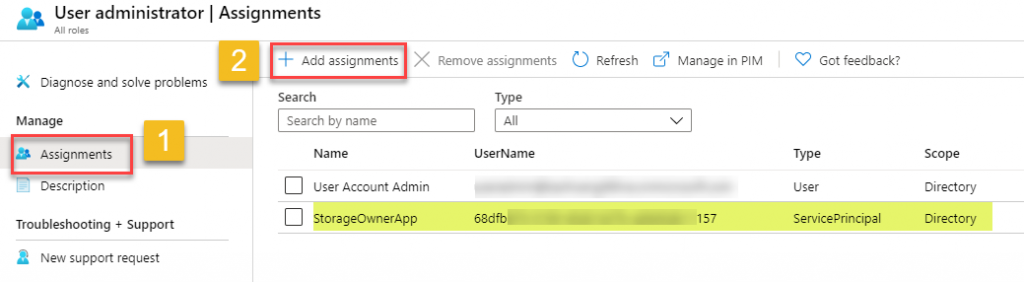
- In order to manage RBAC App Role Assignments, we need to give the Application either the Owner or User Access Administrator RBAC Role at either the resource level or any parent level as documented here. In this example I give the Owner role to this Application at the Azure Storage level since we will be creating an App Role Assignment for the Azure Storage’s Blob Container.
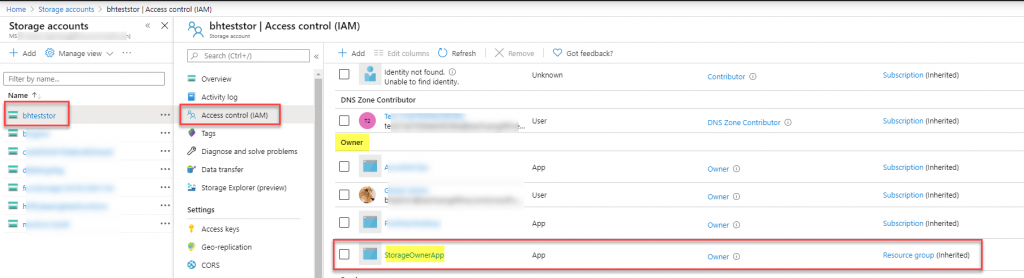
- Get the Role Definition ID for ‘Storage Blob Data Reader’ role. You can use the following Az PowerShell commands to get this:
# Make sure you install the PowerShell Az module before running the following command. See https://docs.microsoft.com/en-us/powershell/azure/new-azureps-module-az?view=azps-3.8.0 for more info. Connect-AzAccount -Tenant "<Tenant Name>" Get-AzRoleDefinition -Name "Storage Blob Data Reader" | Select Name,Id # Here is my my output: <# Name Id ---- -- Storage Blob Data Reader 2a2b9908-6ea1-4ae2-8e65-a410df84e7d1 #>
The Visual Studio Project
- From Visual Studio 2019, create a new C# Console App (.Net Framework)
- Install this Nuget Package: Install-Package Microsoft.Azure.Management.Fluent
- Replace the relevant sections in your Program.cs file with the following code containing your Application Registration info and the ARM path in the Role Assignment section:
[gist id=”c3dd1385e23ceb3f34c9cab163646979″ file=”Program.cs”]
Running the application should produce the following result after creating user, group, and role assignment:
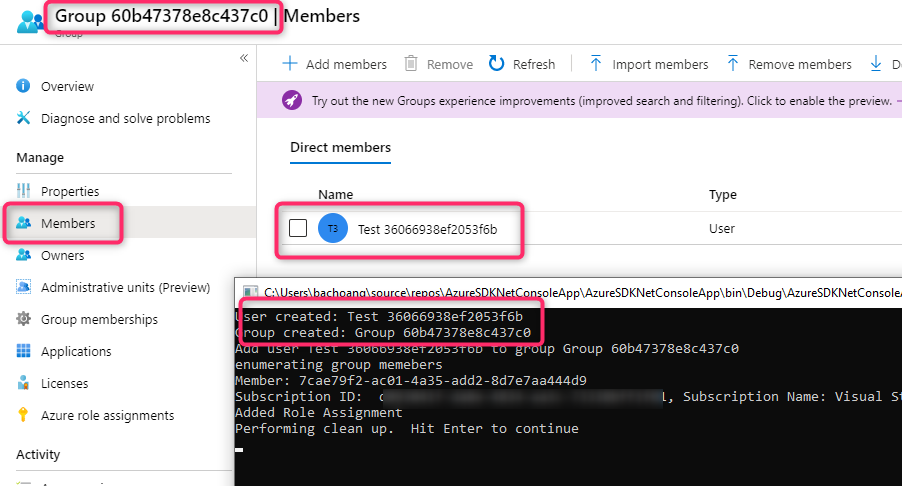
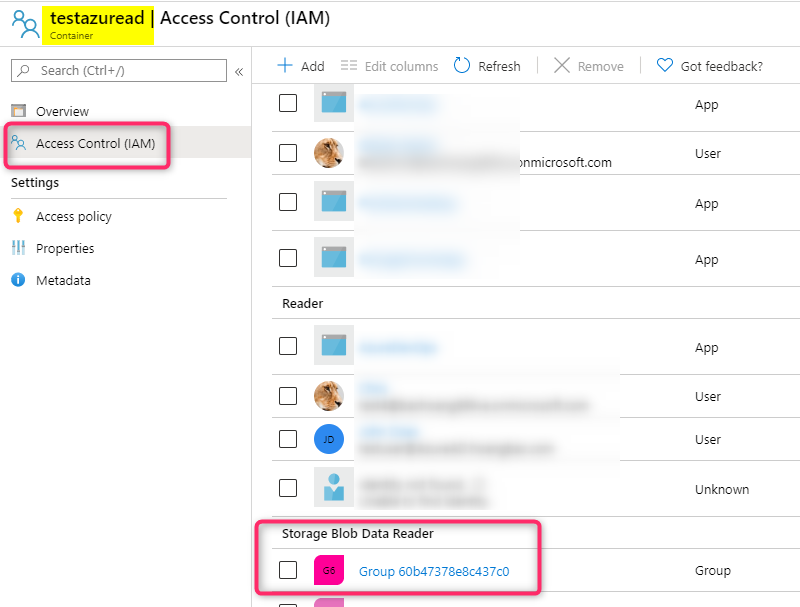
REST endpoint for Role Assignment
If you want to do the above RBAC Role Assignment using Azure Resource Manager (ARM) REST endpoint the request is below:
PUT https://management.azure.com/{scope}/providers/Microsoft.Authorization/roleAssignments/{roleAssignmentName}?api-version=2018-01-01-preview Body: { "properties": { "roleDefinitionId": "/{scope}/providers/Microsoft.Authorization/roleDefinitions/{roleDefinitionId}", "principalId": "{principalId}" } } Note: roleAssignmentName can be any GUID created from GUID Generator tool principalId - this is the Object ID of the Azure AD Directory Object (Group Object ID) roleDefinitionId - this is the RoleDefinitionID from step 4 scope - subscriptions//resourceGroups/ /providers/Microsoft.Storage/storageAccounts/ /blobServices/default/containers/
For other Azure Manage for .Net samples, see https://github.com/Azure/azure-libraries-for-net/tree/master/Samples