When authenticating with Azure and making a Microsoft Graph request, for commercial tenants, this is all done with the .com endpoints ( https://login.micorosoftonline.com/… and https://graph.microsoft.com ) and your token audience is for the .com endpoint as well. However, when performing the requests against a National Cloud tenant, you must use the appropriate endpoints. In this article, I will show you how to configure the Microsoft Graph .Net SDK in a .Net application for use in a US National Cloud.
In this sample, I will be using the Graph Authentication Provider class for the client_credentials grant flow. I previously wrote about this class here. To use the sample, you will need to configure an app registration in your tenant and grant it the Microsoft Graph User.Read.All permission as the sample request will pull a list of users in your tenant. You will also need to configure a client secret since this is required for that flow. You can download the sample here.
In the sample, you can configure the 2 endpoints in the AuthSettings.cs file. The sample is already set for the .us endpoints. You will need to set the tenant_id, the client_id ( app id ) and the client_secret.
namespace GraphServiceClient_ConfidentialClient
{
class AuthSettings
{
public static String instance = "https://login.microsoftonline.us"; // gov tenants would be .us where commercial would be .com
public static String graph_endpoint = "https://graph.microsoft.us"; // gov tenants would be .us where commercial would be .com
public static String graph_version = "v1.0"; // values are v1.0 or beta
public static String tenant_id = ""; // can be the tenant guid or name
public static String client_id = ""; // the app id of the app registration in the tenant
public static String client_secret = ""; // a client secret must be configured on the app registration for the client credentials flow
public static String authority = $"{instance}/{tenant_id}";
// for the client credentials flow, must always use the .default and any application permissions
// consented to will appear in the token. You cannot do dynamic scopes
public static String scope = $"{graph_endpoint}/.default";
public static String graph_baseURL = $"{graph_endpoint}/{graph_version}";
}
}
Compile and run and you should see a list of users from your national cloud tenant like this:
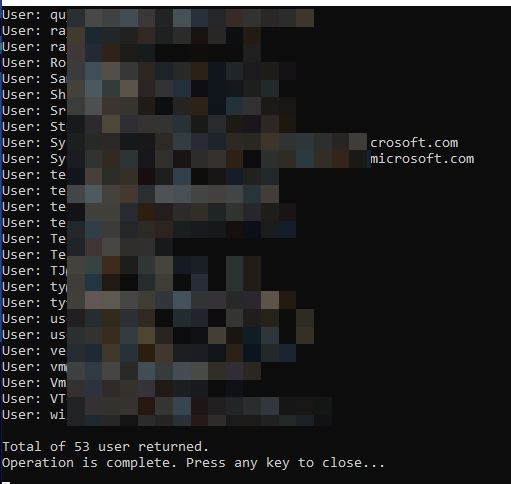
Some key points here. The Scope string is going to use the .us endpoint from the graph_endpoint value. If you do not provide a scope, the Graph Service Client will default to the .com endpoint. Also, you will see that in the Program.cs file, in the Get_Users method, line 42, I specify the base url endpoint. If you do not provide the base url, the Graph Service Client will default to the .com endpoint. These are the 2 main sticking points for national cloud tenants as they try to use the graph client on their tenant.
And that is it!. There are only a couple of things that must be changed to get the graph client to point to your national cloud endpoint.